2.2. If Statements#
The if
statement lets us execute a section of code if a specified condition is True.
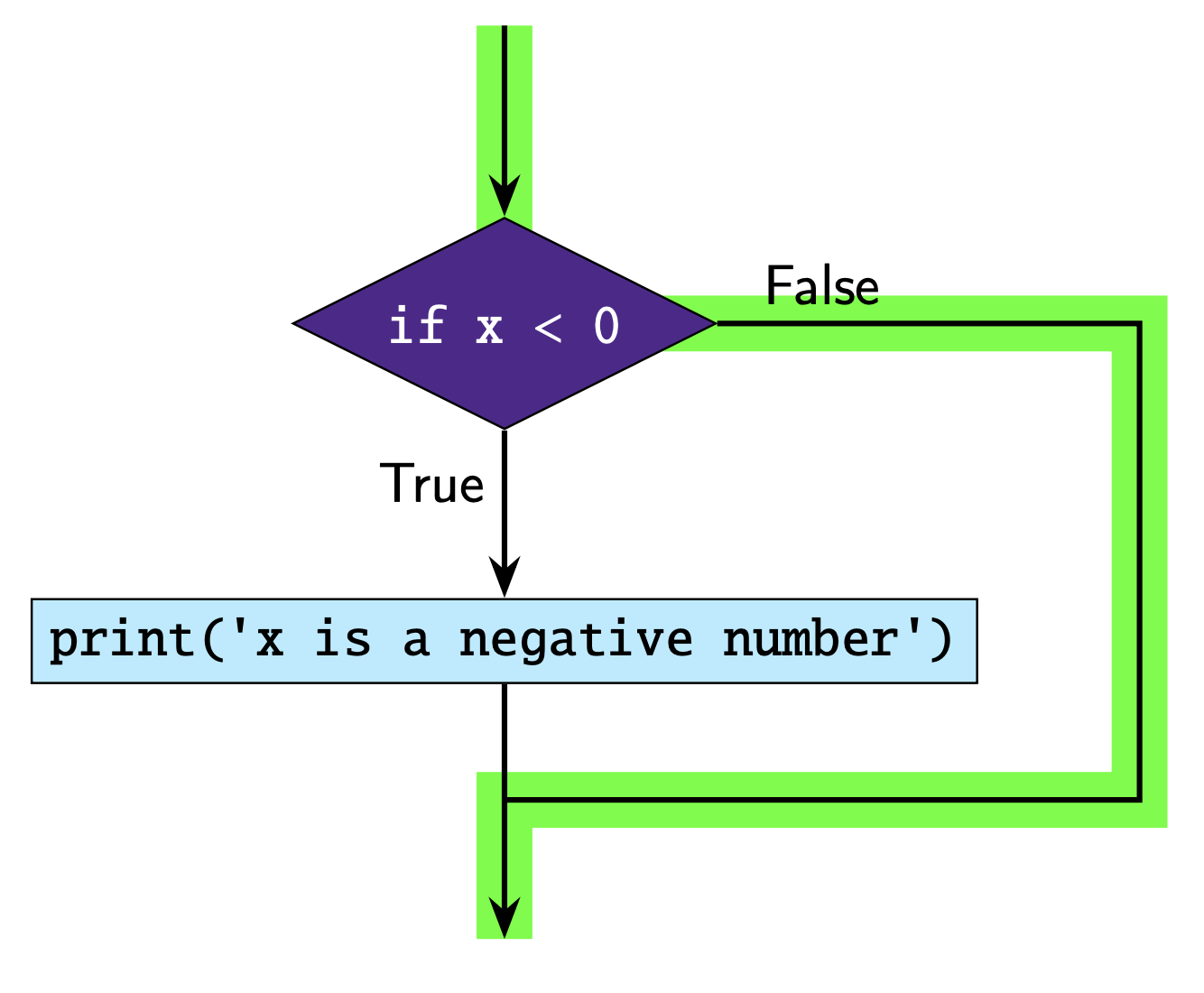
The structure of an if statement is:
if condition:
# code you execute if condition is true
Take note of the following:
if
is a keywordThe condition must evaluate to either
True
orFalse
The code inside the if statement only executes if the condition is
True
:
is placed at the end of the conditionThe code inside the if statement must be indented. The indentation defines the code block. This allows you to put multiple lines inside the if statement.
The indentation can be done using tab or spaces, as long as you’re consistent!
Here is an example of a simple if statement.
x = -2
if x < 0:
print('x is a negative number')
x is a negative number
In this example the condition is True
so the print statement runs.
This is how we can represent this code diagrammatically. The green line indicates the ‘path’ the code takes.
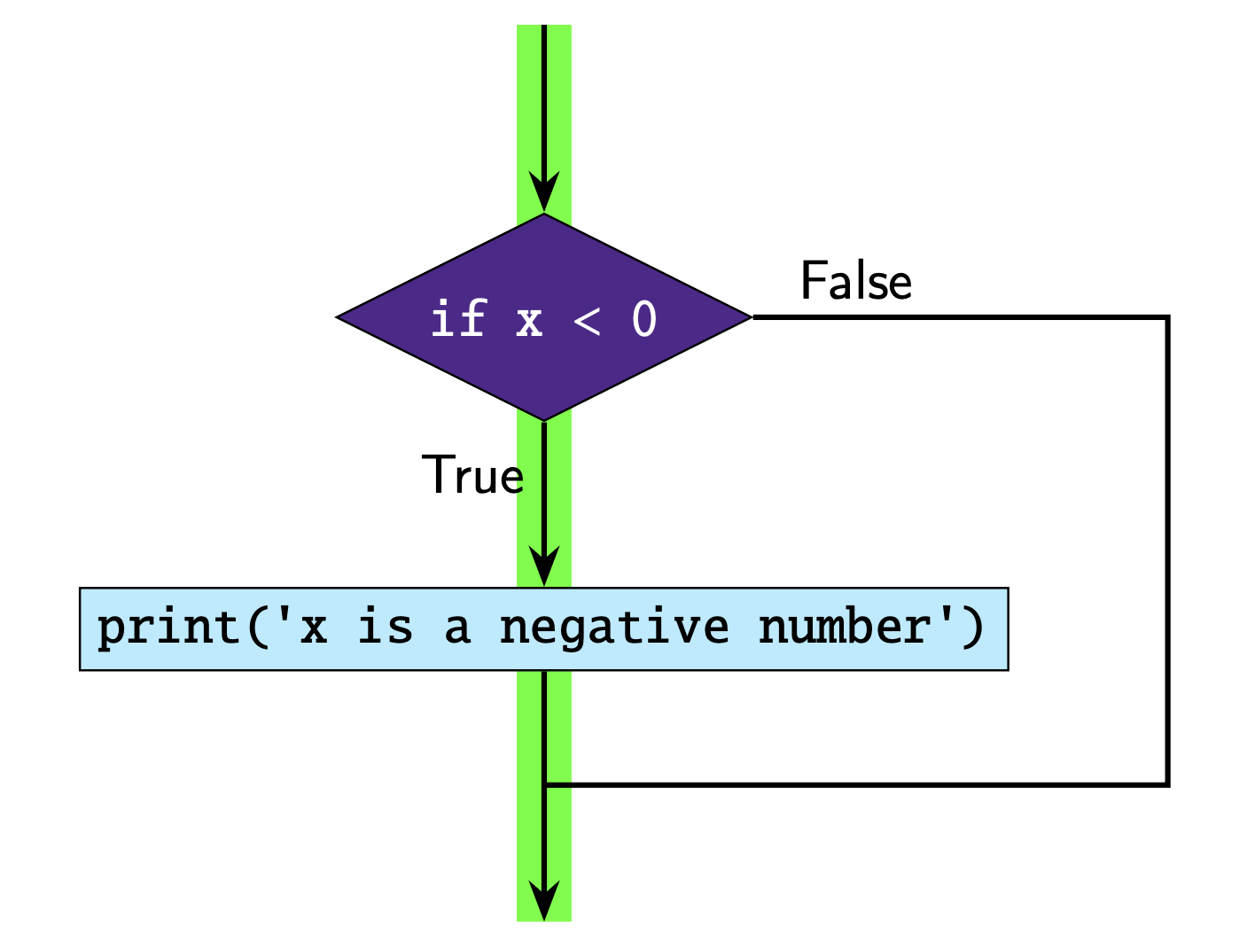
Let’s look at another example.
x = 1
if x < 0:
print('x is a negative number')
In this example the condition is False
so the print statement does not run.
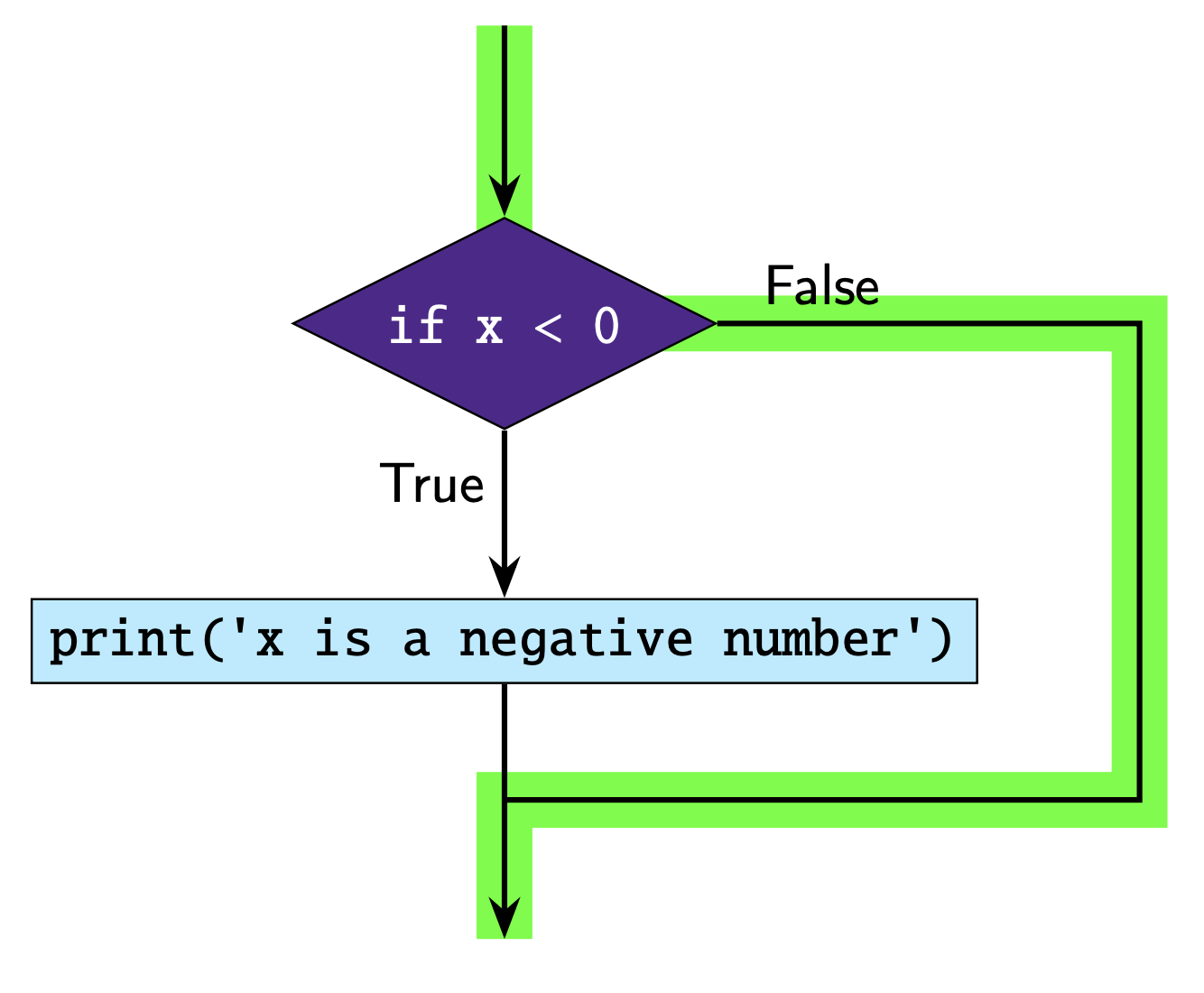